Intro
This is the object layer sub page of the example. If you are new to the series, click here to access the main page first.
Map setup
- Create an object layer and give it a name, e.g. “Collisions”.
- Draw your collisions with the object tools. Hold ctrl to snap to corners.
Comparison
Object layer
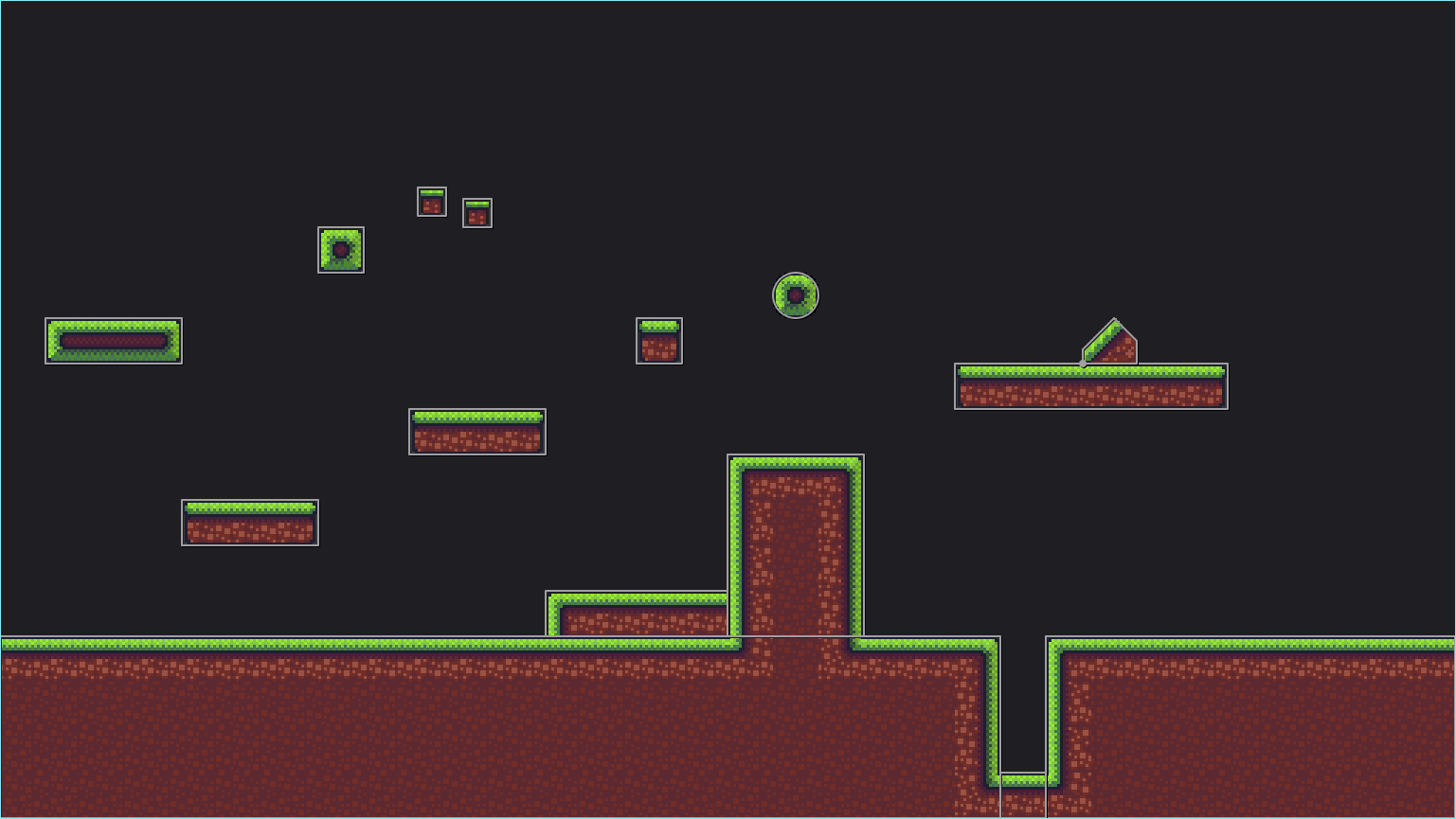
Tile collisions
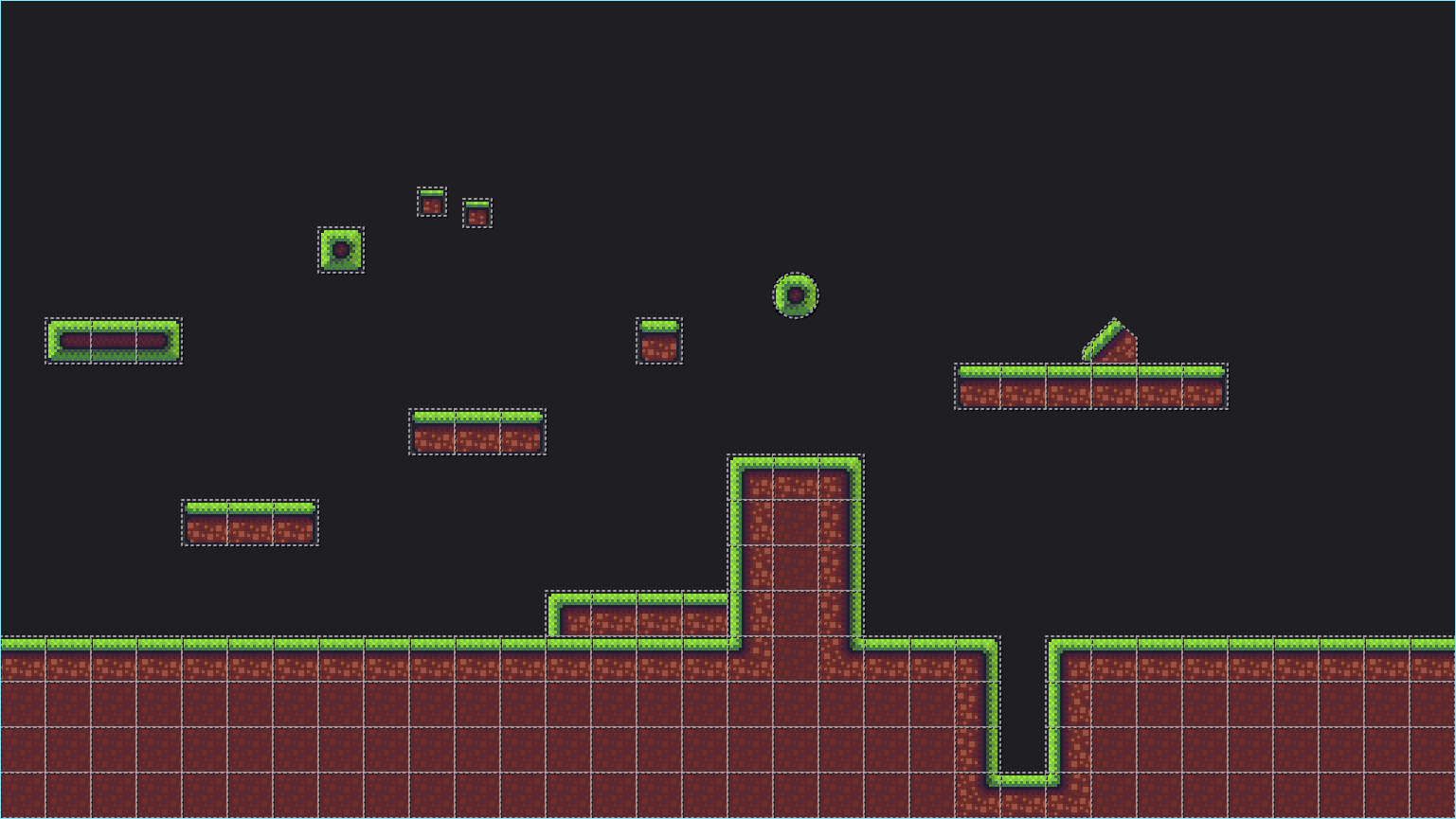
As you see, the object layer version creates a lot less rectangles, therefore is faster to parse and might work better. The tile collision version however is automated and you don’t need to care about creating collisions shapes.
Implementation
Now for the fun part!
Let’s iterate through the objects.
1 | MapObjects collisions = map.getLayers().get("Collisions").getObjects(); |
Then we’ll need to get the actual shape in the map object.
This is done by checking which class it actually is (then converting to it), and calling .getXXX()
on it.
1 | if (mapObject instanceof RectangleMapObject) |
Box2d body creation
Now inside each if …
Rectangle
In Tiled every object’s x/y coordinates are bottom left. Sadly Box2D uses center coordinates. Therefore we’ll need to add half the width/height to the position.
1 | if (mapObject instanceof RectangleMapObject) { |
.setAsBox()
accepts half width/height parameters, therefore we’ll need to half the width/height.
1 | Body body = world.createBody(bodyDef); |
Circle
Here we have another little problem, Tiled allows ellipses, Box2D only circles. Therefore we’ll need to check if the ellipse is actually a circle.
Additionally ellipse coordinates are already centered, so we don’t have that problem here.
1 | else if (mapObject instanceof EllipseMapObject) { |
Then we’ll check if it’s a circle, if not we’ll exit.
1 | if (ellipse.width != ellipse.height) |
1 | Body body = world.createBody(bodyDef); |
Polygon
Polygons are probably the most easiest. However you can only load polygons with a maximum of 8 points.
1 | else if (mapObject instanceof PolygonMapObject) { |
That’s pretty much everything you need!